Recently I wanted to do some cleanup and re-organization in Azure DevOps (ADO) project. Removed some teams, reviewed branch policies etc. During this cleanup I saw those long living development branches in the repositories. Normally, when a Pull Request (PR) is completed, ADO gives an option to delete source branch when the PR is completed.
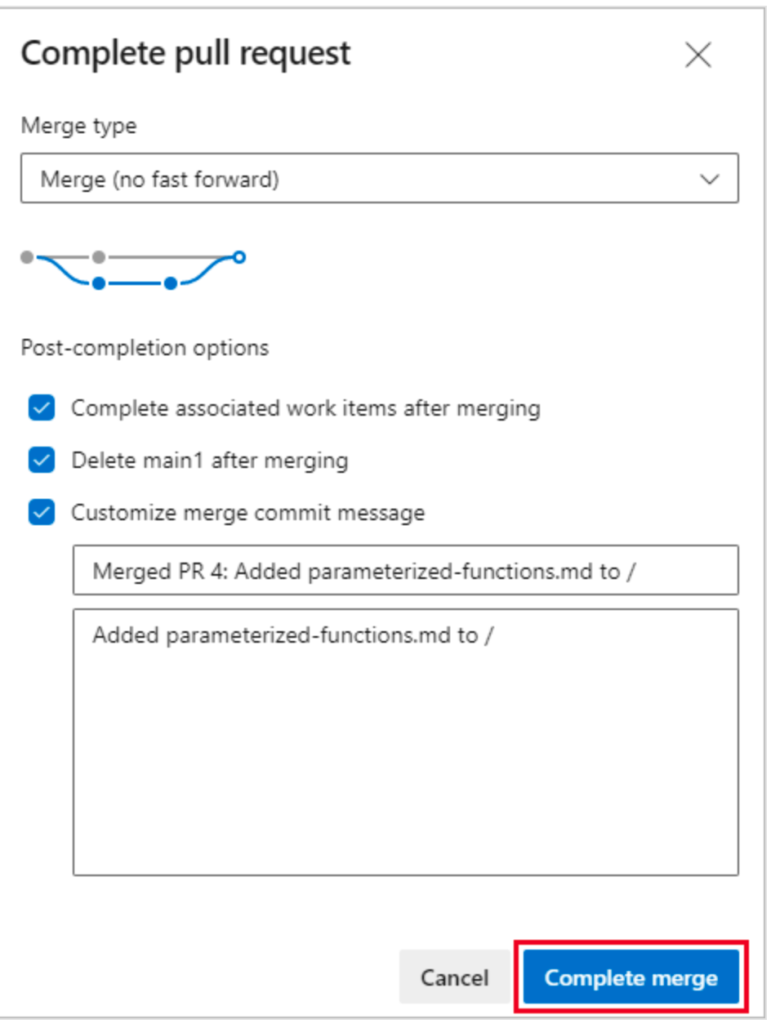
However, sometimes developers do not click this option. Sometimes they create a branch, push it to remote but then forget about it. There are many reasons branches are created and then remain there for a long time. If it is few, then I go an delete them one by one. However in the last project, there were over 20 repositories and removing them manually did not make any sense. On top of that, they would grow again instantly. Therefore I decided to do some automation for the operation.
Pipelines are the best
I decided to create a pipeline which would go through each repository, then do some operation for me. So I made my plan as;
- Go through all the repositories in selected project
- Find all short living branches (I exclude main/master/release branches because those are long living branches)
- Check if there are any open pull request for this branch. If so, then skip
- Check if there are any commit in the last 2 weeks for this branch. If so, then skip.
- Delete the branch.
- Continue
Idea was simple. Couple of iterations, some simple API calls and lots of validations. I wanted to use ADO Rest APIs and PowerShell script to write my code.
# Azure DevOps Organization and Project Details
$organization = "https://dev.azure.com/myOrganization"
$project = "myProject"
$accessToken = "myPAT"
Write-Host "Fetching repositories from project '$project'..."
# Authorization Header
$headers = @{
Authorization = "Bearer $accessToken"
}
After preparing the pre-requisities, I started fetching the data.
# Get Repositories
$reposUrl = "$organization/$project/_apis/git/repositories?api-version=7.2-preview.1"
$repositories = (Invoke-RestMethod -Uri $reposUrl -Headers $headers).value
Write-Host "Found $($repositories.Count) repositories."
foreach ($repo in $repositories) {
$repoId = $repo.id
if (-not $repoId) {
Write-Warning "Repository '$($repo.name)' does not have a valid ID. Skipping."
continue
}
Write-Host "Processing repository: $($repo.name) ($repoId)..."
$branchesUrl = "$organization/$project/_apis/git/repositories/$repoId/refs?filter=heads/&api-version=7.2-preview.1"
$branches = (Invoke-RestMethod -Uri $branchesUrl -Headers $headers).value
foreach ($branch in $branches) {
$branchName = $branch.name -replace "refs/heads/", ""
Write-Host "Checking branch: $branchName..."
if ($branchName -notin @("main", "release", "master")) {
# Check for open pull requests
$prUrl = "$organization/$project/_apis/git/repositories/$repoId/pullRequests?searchCriteria.status=active&searchCriteria.sourceRefName=refs/heads/$branchName&api-version=7.2-preview.2"
$prs = (Invoke-RestMethod -Uri $prUrl -Headers $headers).value
if ($prs.Count -gt 0) {
Write-Host "Branch '$branchName' has open pull requests and was skipped."
continue
}
Write-Host "Branch '$branchName' does not have any open pull requests"
if ($prs.Count -eq 0) {
# Check last commit date
$commitsUrl = "$organization/$project/_apis/git/repositories/$repoId/commits?searchCriteria.itemVersion.version=$branchName&searchCriteria.$top=1&api-version=7.2-preview.2"
$lastCommit = (Invoke-RestMethod -Uri $commitsUrl -Headers $headers).value[0]
if (-not $lastCommit) {
Write-Warning "Branch '$branchName' has no commits. Skipping."
continue
}
if ($lastCommit) {
$commitDate = [datetime]$lastCommit.author.date
Write-Host "Last commit on branch '$branchName' was on $commitDate."
if ((Get-Date) - $commitDate -le 14) {
Write-Host "Branch '$branchName' has recent commits. Skipping."
ontinue
}
if ((Get-Date) - $commitDate -gt 14) {
# Try to delete branch
$lastCommitId = $lastCommit.commitId
Write-Host "Attempting to delete branch: $branchName..."
Write-Host "Last commit id is $lastCommitId"
try {
$deleteUrl = "$organization/$project/_apis/git/repositories/$repoId/refs?api-version=6.1-preview.1"
$payload = @(
@{
name = "refs/heads/$branchName"
oldObjectId = $lastCommitId
newObjectId = "0000000000000000000000000000000000000000"
}
) | ConvertTo-Json -Depth 10 -Compress
$payload = "[$payload]"
$response = Invoke-RestMethod -Uri $deleteUrl -Method Post -Headers $headers -Body $payload -ContentType "application/json"
Write-Host "Response: $response | ConvertTo-Json -Depth 10"
if ($response.value[0].success -eq $true) {
Write-Host "Deleted branch: $branchName"
} else {
Write-Warning "Failed to delete branch '$branchName': $($response | ConvertTo-Json -Depth 10)"
}
catch {
$responseContent = $null
if ($_.Exception.Response -ne $null -and $_.Exception.Response.Content -ne $null) {
try {
# Access content before it's disposed
$responseContent = $_.Exception.Response.Content.ReadAsStringAsync().Result
} catch {
Write-Warning "Failed to read error response content: $($_.Exception.Message)"
}
}
Write-Warning "Failed to delete branch '$branchName': $($_.Exception.Message)"
if ($responseContent) {
Write-Warning "Detailed error response: $responseContent"
}
}
}
}
} else {
Write-Host "Branch '$branchName' has open pull requests and was skipped."
}
}
}
}
I must say figuring out the working code was quite a challenge. Many trial-errors but finally I made it working. There are of course some things I had figured out after some research and lots of documentation reading.
- There is no endpoint to delete a branch. According to microsoft documentation, to delete a branch you need to update the last commit of a ref to “0000000000000000000000000000000000000000”. Then ADO will consider this branch deleted. For the documentation https://learn.microsoft.com/en-us/rest/api/azure/devops/git/refs/update-refs?view=azure-devops-rest-7.1&tabs=HTTP
- Payload of updating refs requires an object in an array. Initially I just sent the payload as following
POST https://dev.azure.com/fabrikam/_apis/git/repositories/{repositoryId}/refs?api-version=7.1
{
"name": "refs/heads/vsts-api-sample/answer-woman-flame",
"oldObjectId": "0000000000000000000000000000000000000000",
"newObjectId": "ffe9cba521f00d7f60e322845072238635edb451"
}
However this failed with an error message. After some review I noticed the documentation has a sample post method and there I figured out the array brackets. After adding the brackets, the issue is resolved.
Increasing the security
My code was working, but I was not happy with storing the PAT in the code as it is. Therefore I wanted to store it in a Key Vault, and read it from there during the run. For that, I added my PAT as a secret to Key Vault and updated my pipeline code accordingly.
steps:
- task: AzureKeyVault@2
displayName: "Fetch Token"
inputs:
azureSubscription: "mySubscription"
KeyVaultName: "myKeyVaultName"
SecretsFilter: "delete-branch-pipeline-token"
RunAsPreJob: false
- task: PowerShell@2
displayName: "Delete Stale Branches"
inputs:
targetType: "inline"
script: |
# Azure DevOps Organization and Project Details
$organization = "https://dev.azure.com/myOrganization"
$project = "myProject"
Write-Host "Fetching repositories from project '$project'..."
# Authorization Header
$headers = @{
Authorization = "Bearer $(delete-branch-pipeline-token)"
}
That way my PAT was not stored in source code and could not be seen during the pipeline run either.